快速开始
大约 3 分钟基础知识教程快速开始
本教程将会指引你如何使用Fantasy 3D开发项目。
安装
npm
// 安装核心包
npm i @fantasy3d/core
// 安装插件包
npm i @fantasy3d/addons
yarn
// 安装核心包
yarn add @fantasy3d/core
// 安装插件包
yarn add @fantasy3d/addons
注意
由于three.js被当做peerDependencies依赖,而yarn不会自动安装peerDependencies中的依赖, 所以需要手动安装three.js依赖且版本需要>=0.168.0。
yarn add three@0.168.0
使用
- 设置渲染视口
在HTML中插入一个div标签:
<div id="viewport" style="width: 100%; height: 100%"></div>
注意
确保 div 元素的宽度和高度大于零,以确保其内容能够正确渲染!
- 创建引擎
import { Engine } from '@fantasy3d/core';
// 创建引擎
const engine = new Engine( {
// WebGL Options
gl: {
viewport: document.getElementById( 'viewport' ), // 渲染视口
clearColor: 'orange' // 背景颜色
}
} );
- 创建场景
import { Scene, SceneRenderer } from '@fantasy3d/core';
// 创建场景
const scene = new Scene( engine );
// 设置场景渲染器
scene.sceneRenderer = new SceneRenderer( engine );
// 激活场景
scene.isActive = true;
- 创建相机
import { Vector3 } from 'three';
import { OrbitController } from '@fantasy3d/addons';
// 创建一个透视相机
const camera = scene.createPerspectiveCamera( {
// 相机方位属性
transform: {
position: new Vector3( 0.0, 5.0, 5.0 ),
lookAt: new Vector3( 0.0, 0.0, 0.0 )
},
// 相机属性
camera: { near: 0.1, far: 100.0 }
} );
// 添加一个相机控制器组件
camera.addComponent( OrbitController, { damping: true } );
- 添加灯光
import { AmbientLight, DirectionalLight } from '@fantasy3d/core';
// 获取场景根实体
const { rootEntity } = scene;
// 添加一个环境光到场景根实体
rootEntity.addComponent( AmbientLight, { intensity: 0.25 * Math.PI } );
// 添加一个方向光到场景根实体
rootEntity.addComponent( DirectionalLight, {
intensity: Math.PI,
position: new Vector3( 20.0, 20.0, 20.0 ),
target: new Vector3( 0.0, 0.0, 0.0 )
} );
- 创建一个立方体
import { BoxGeometry, Euler, Mesh, MeshLambertMaterial, Vector3 } from 'three';
import { MeshRenderer } from '@fantasy3d/core';
// 创建一个子实体
const entity = rootEntity.createChild( {
transform: {
position: new Vector3( 0.0, 2.0, 0.0 ),
rotation: new Euler( 0.0, Math.PI / 4.0, 0.0 )
}
} );
// 添加一个MeshRenderer组件
entity.addComponent( MeshRenderer, {
mesh: new Mesh(
new BoxGeometry( 4.0, 4.0, 4.0 ),
new MeshLambertMaterial( { color: 'lightblue' } )
)
} );
完整代码:
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, user-scalable=no, minimum-scale=1.0, maximum-scale=1.0">
<title>Quick Start</title>
<style>
html {
height: 100%;
}
body
{
font-family: Monospace;
font-weight: bold;
margin: 0px;
height: 100%;
overflow: hidden;
}
</style>
</head>
<body>
<div id="viewport" style="width:100%; height:100%"></div>
<script src="./js/loadscripts.js"></script>
<script>loadscripts( {
"@fantasy3d/core": "../dist/fantasy.core.js",
"@fantasy3d/addons": "../dist/fantasy.addons.js"
} )</script>
<script type="module">
import { BoxGeometry, Euler, Mesh, MeshLambertMaterial, Vector3 } from 'three';
import { AmbientLight, DirectionalLight, Engine, MeshRenderer, Scene, SceneRenderer } from '@fantasy3d/core';
import { OrbitController } from '@fantasy3d/addons';
// 创建引擎
const engine = new Engine( {
// WebGL Options
gl: {
viewport: document.getElementById( 'viewport' ), // 渲染视口
clearColor: 'orange' // 背景颜色
}
} );
// 创建场景
const scene = new Scene( engine );
// 设置场景渲染器
scene.sceneRenderer = new SceneRenderer( engine );
// 激活场景
scene.isActive = true;
// 创建一个透视相机
const camera = scene.createPerspectiveCamera( {
// 相机方位属性
transform: {
position: new Vector3( 0.0, 5.0, 5.0 ),
lookAt: new Vector3( 0.0, 0.0, 0.0 )
},
// 相机属性
camera: { near: 0.1, far: 100.0 }
} );
// 添加一个相机控制器组件
camera.addComponent( OrbitController, { damping: true } );
// 获取场景根实体
const { rootEntity } = scene;
// 添加一个环境光到场景根实体
rootEntity.addComponent( AmbientLight, { intensity: 0.25 * Math.PI } );
// 添加一个方向光到场景根实体
rootEntity.addComponent( DirectionalLight, {
intensity: Math.PI,
position: new Vector3( 20.0, 20.0, 20.0 ),
target: new Vector3( 0.0, 0.0, 0.0 )
} );
// 创建一个子实体
const entity = rootEntity.createChild( {
transform: {
position: new Vector3( 0.0, 2.0, 0.0 ),
rotation: new Euler( 0.0, Math.PI / 4.0, 0.0 )
}
} );
// 添加一个MeshRenderer组件
entity.addComponent( MeshRenderer, {
mesh: new Mesh(
new BoxGeometry( 4.0, 4.0, 4.0 ),
new MeshLambertMaterial( { color: 'lightblue' } )
)
} );
</script>
</body>
</html>
提示
loadscripts.js文件实现代码如下:
const version = "0.161.0";
const threeImportmap = {
"three": `https://unpkg.com/three@${version}/build/three.module.js`,
"three/examples/jsm/": `https://unpkg.com/three@${version}/examples/jsm/`
}
function loadscripts( inputImports ) {
// Import maps polyfill
// Remove this when import maps will be widely supported
const script_shims = document.createElement( 'script' );
script_shims.async = true;
script_shims.src = './js/libs/es-module-shims.js';
document.body.appendChild( script_shims );
const imports = Object.assign( inputImports, threeImportmap );
const scirpt_importmap = document.createElement( 'script' );
scirpt_importmap.type = 'importmap';
scirpt_importmap.innerHTML = `{
"imports": ${JSON.stringify( imports )}
}`;
document.body.appendChild( scirpt_importmap );
}
最终效果:
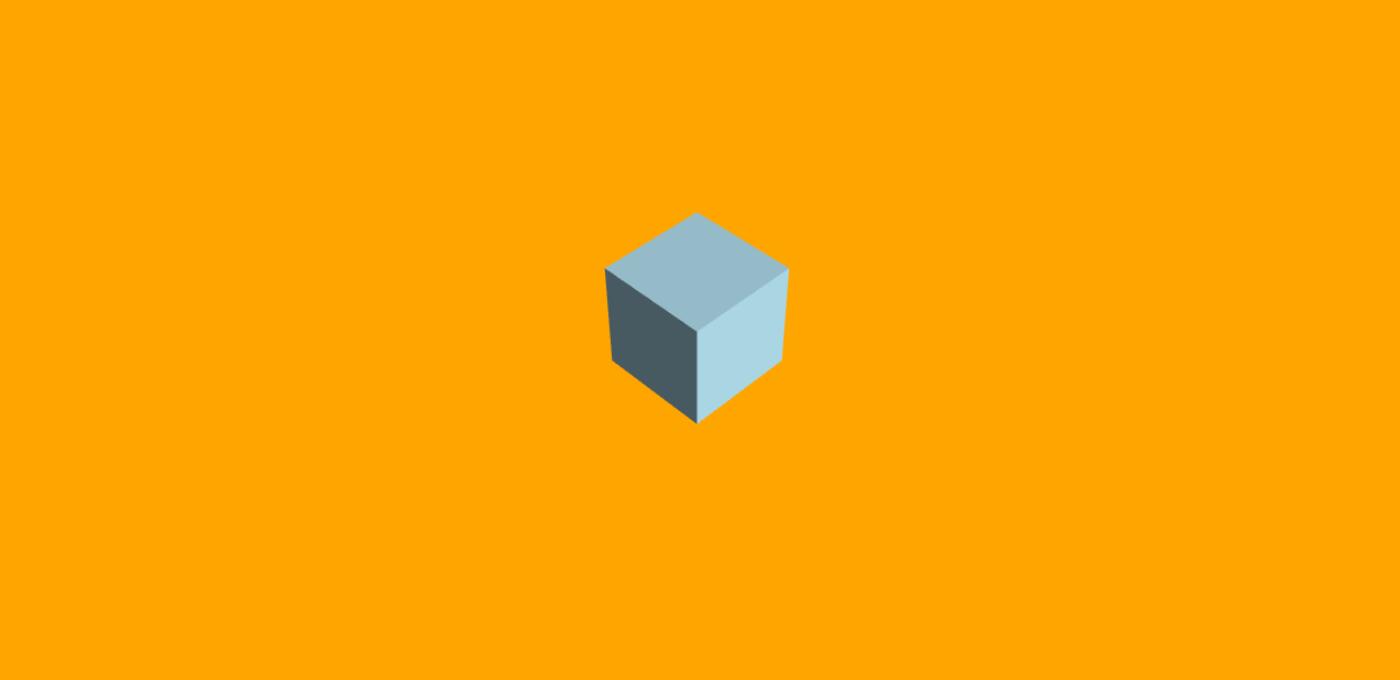