脚本
自定义脚本的基类是 Script,它继承自 Component。通过在自定义脚本的生命周期回调函数中编写的逻辑代码,可以扩展引擎功能。
提示
脚本生命周期回调函数不需要手动调用,Fantasy 3D会在特定的时期自动调用相关回调函数。
脚本生命周期
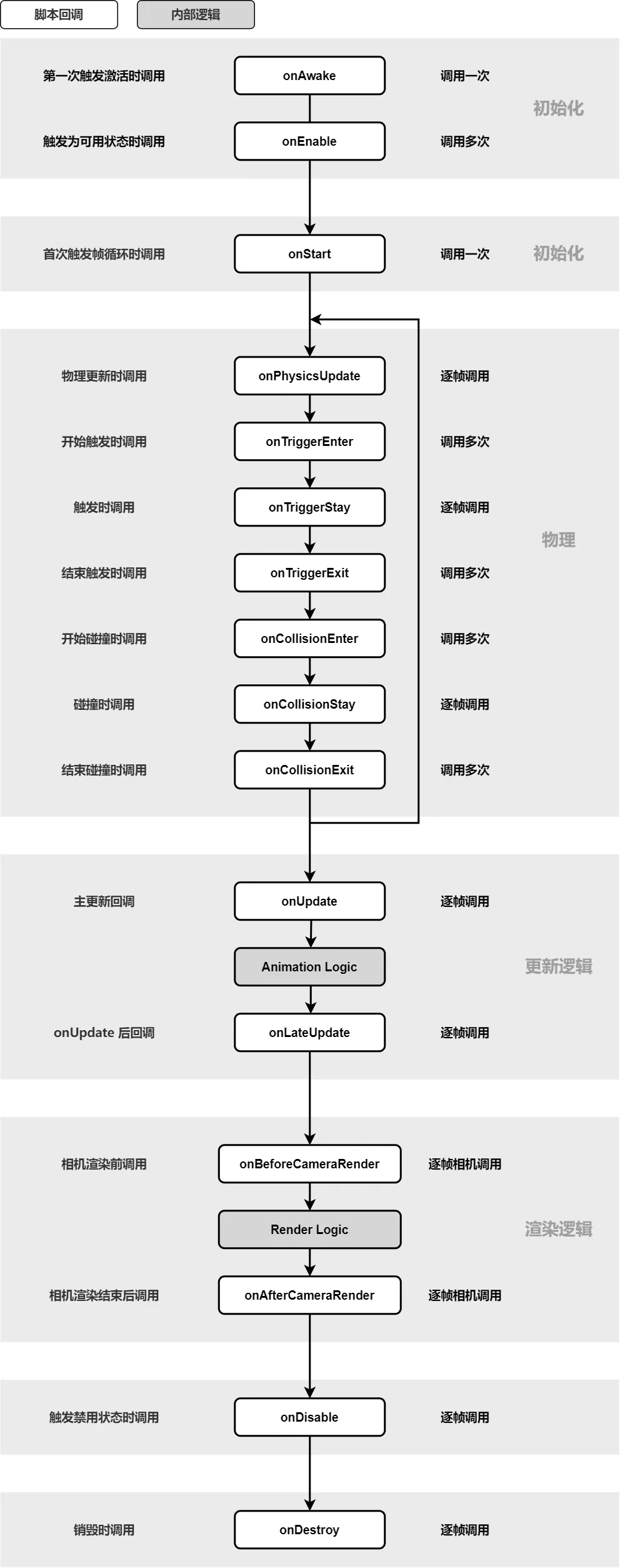
添加脚本时如果实体的 activeInHierarchy 为 true, 则在脚本初始化时回调函数将被调用,如果 activeInHierarchy 为 false,则在实体激活时,即 setActive( true ) 时被调用。onAwake 只会被调用一次,且在所有生命周期的最前面。
当脚本的 enabled 属性设置为 true 时,或者调用所在实体的 setActive( true ) 时,会调用 onEnable 回调。
当脚本的 enabled 属性设置为 false 时,或者调用所在实体的 setActive( false ) 时,会调用 onDisable 回调。
onStart 回调函数会在脚本第一次进入帧循环,也就是第一次执行 onUpdate 之前触发。
onPhysicsUpdate 回调函数调用频率与物理引擎更新频率保持一致。每个渲染帧可能会调用多次。
onTriggerEnter 回调函数在触发器相互接触时调用,只调用一次。
onTriggerStay 回调函数在触发器接触过程中持续调用。
onTriggerExit 回调函数在触发器分离时调用,只调用一次。
onCollisionEnter 回调函数在碰撞器碰撞时调用,只调用一次。
onCollisionStay 回调函数在碰撞器碰撞过程中持续调用。
onCollisionExit 回调函数在碰撞器分离时调用,只调用一次。
onUpdate 回调函数在动画更新逻辑之前调用,逐帧调用。
onLateUpdate 回调函数在动画更新逻辑之后调用,逐帧调用。
onBeforeCameraRender 回调函数在渲染逻辑之前调用,逐相机调用。
onAfterCameraRender 回调函数在渲染逻辑之后调用,逐相机调用。
当组件或所在实体调用了 destroy,则会调用 onDestroy 回调函数。
自定义脚本
import { Entity, Script, defaultValue } from '@fantasy3d/core';
/**
* 定义绕Y轴旋转脚本
*/
class RotateScript extends Script {
/**
* 旋转速度
*/
speed: number = 1.0;
constructor( entity: Entity, parameters = {} ) {
super( entity, parameters );
this.speed = defaultValue( parameters.speed, 1.0 );
}
// public functions
/**
* 帧更新回调
*/
onUpdate( timeSinceLastFrame: number, totalTime: number ): void {
const { entity, speed } = this;
const { transform } = entity;
// 使用 three.js 原生 Object3D 对象更新 Y 轴旋转量
transform.object3D.rotation.y += speed * timeSinceLastFrame;
// 由于使用 three.js 原生对象更新旋转量,所以此处必须手动更新世界矩阵
transform.updateWorldMatrix();
}
}
// 使用旋转脚本组件
.........
entity.addComponent( RotateScript, { speed: 2.0 } );
.........